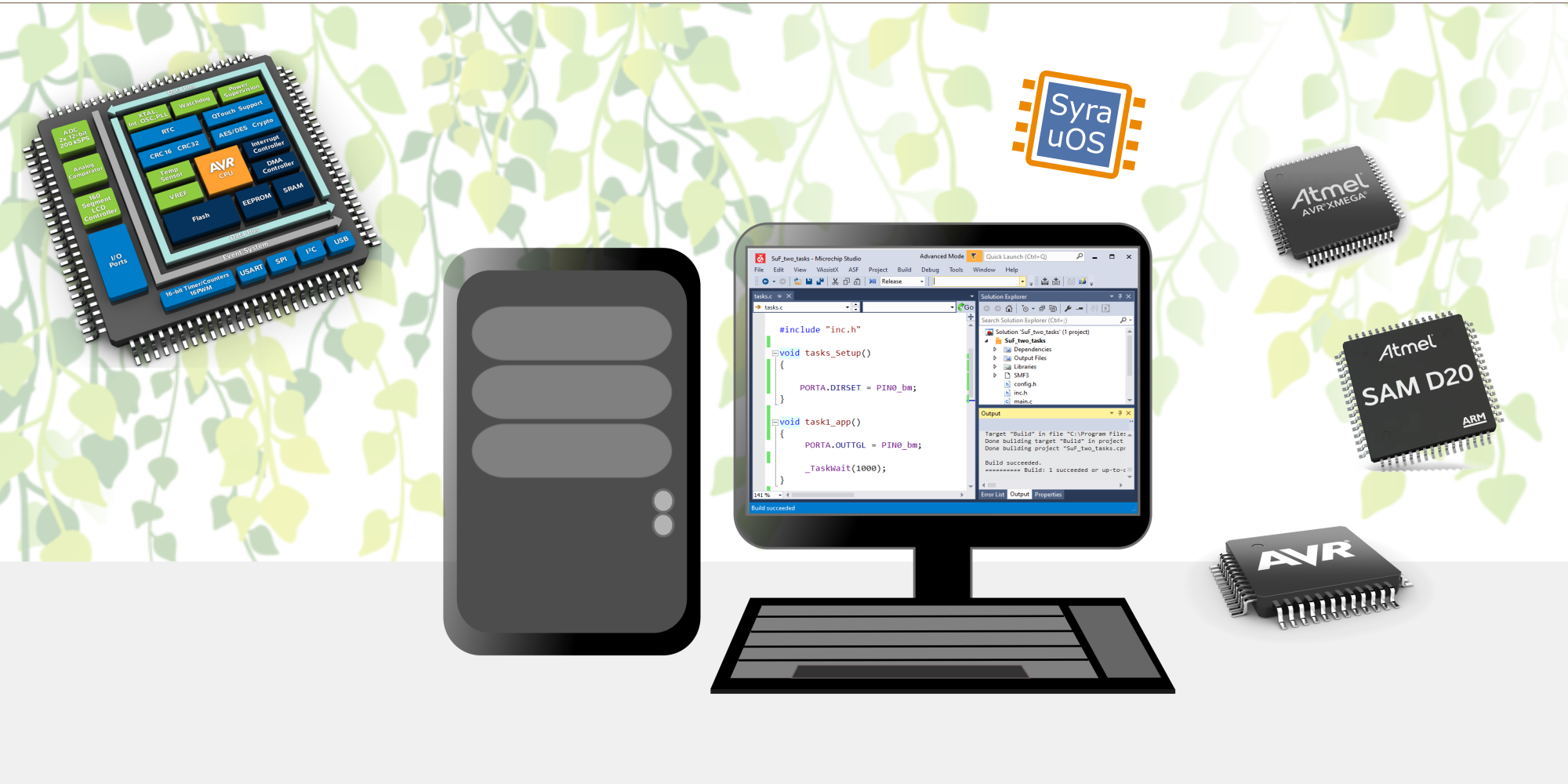
Syra uOS
A framework library that helps you write your powerful code for microcontrollers.
Getting started with Syra uOS
It is an asynchronous event-driven framework library, work as a tiny operating system for microcontrollers. It is designed to build scalable microcontroller applications. In the following example, we’ll show how to create a simple task.
A Simple Task
Task is defined as a normal function, but it does not take any input data. Also, It does not return any data.
The statements inside the task being executed repeatedly as any infinite loop.
This example for an XMega AVR Microcontroller. This task changes the status of PIN0 of PORTA , then it waits for 1000ms before next execution.
void task1_app() { // toggle the status of pin 0 PORTA_OUTTGL = PIN0_bm; // wait for 1sec before next execution TaskWait(1000); }
Setup The Task
Before start executing the task there is a need to do some configurations or define some variables and set their initial values.
Here is an example of Setup function that set the direction of PIN0 of PORTA as an output.
This setup function will be executed before running the task in the system loop.
void task1_Setup() { // Define Pin 0 of Port A as output PORTA_DIRSET = PIN0_bm; }
Register The Task
To run your task, you need to register it in the system.
The best place to register your task is in the OnBoot method. because the framework executes this method before running tasks.
also, in this method we call all setup functions for all tasks.
Registering task is done by calling the method Sys_CreateTask.
As in the example we pass the task name as a parameter.
If you need to execute additional tasks just repeat the previous steps.
/* // This section is part of the file main.c // */ void OnBoot() { // calling the setup function task1_Setup(); // Registering Task 1 in the system Sys_CreateTask(task1_app); // Registering additional tasks Sys_CreateTask(task2_app); Sys_CreateTask(task3_app); Sys_CreateTask(task4_app); . . . }
Supported Devices
tinyAVR®0-series:
ATtiny204, ATtiny404, ATtiny804, ATtiny1604, ATtiny406, ATtiny806, ATtiny1606, ATtiny807, ATtiny1607
tinyAVR®1-series:
Ttiny212, ATtiny412, ATtiny214, ATtiny414, ATtiny814, ATtiny1614, ATtiny416, ATtiny816, ATtiny1616, ATtiny3216, ATtiny417, ATtiny817, ATtiny1617, ATtiny3217
tinyAVR®2-series:
ATtiny424, ATtiny824, ATtiny1624, ATtiny3224, ATtiny426, ATtiny826, ATtiny1626, ATtiny3226, ATtiny427, ATtiny827, ATtiny1627, ATtiny3227
AVR XMega Devices:
ATXMEGA128A1, ATXMEGA128A1, ATXMEGA128A1U, ATXMEGA128A3, ATXMEGA128A3U, ATXMEGA128A4U, ATXMEGA128D4, ATXMEGA16A4, ATXMEGA16A4U, ATXMEGA16D4, ATXMEGA192A3, ATXMEGA192A3U, ATXMEGA256A3, ATXMEGA256A3U, ATXMEGA32A4, ATXMEGA32A4U, ATXMEGA32D4, ATXMEGA64A1, ATXMEGA64A1U, ATXMEGA64A3, ATXMEGA64A3U, ATXMEGA64A4, ATXMEGA64A4U, ATXMEGA64D4,
SAMD ARM® Cortex®-M0+ Devices:
ATSAMD20E14A, ATSAMD20E14A, ATSAMD20E14B, ATSAMD20E15A, ATSAMD20E15B, ATSAMD20E16A, ATSAMD20E16B, ATSAMD20E17A, ATSAMD20E18A, ATSAMD21E15B, ATSAMD21E15L, ATSAMD21E16B, ATSAMD21E16L, ATSAMD21E17A, ATSAMD21E17D, ATSAMD21E17L, ATSAMD21E18A,